Create Apps using Blogger API in Flutter
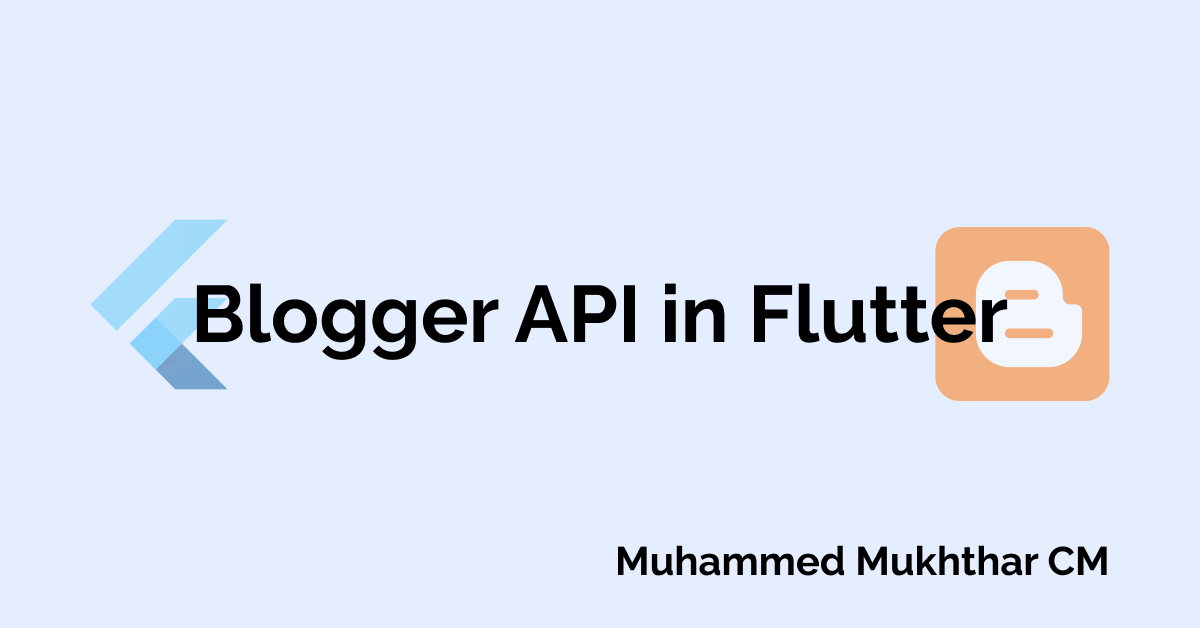
I remember using blogger for starting my First Blog. For sure that was one of my First personal spaces on Internet.
You May have noticed that I am currently using Hugo for generating my Website.
And a fantastic open source theme named hugo-PaperMod
created by adityatelange.
I use hugo because it’s a fantastic platform. Themes like these make life easier for persons like me, who don’t know much of Web dev.
So let’s get in to the point.
Things Needed
For creating an App using Blogger API, you’ll have to know Blogger APi Key
and Blogger Blog Id
.
The easiest way for creating an API key to go here, and Click on Get A Key
Button.
Then Select a Google Clound Project from Popup or create a new one. Don’t forget to take note of the API Key you get now 😬.
The Blog ID can be Identified by going to Blogger.com and looking at the url.
Let’s move to the coding part.
Coding Time
First of All, create a new Flutter project, if you don’t have one already.
flutter create blogger-api-app
For creating the Model class, I Used Json to Dart convertor over here. For this I had to get an Idea of How the blogger API response will look like. So I referred to blogger api documentation, and found the structure to be like this.
{
"kind": "blogger#postList",
"nextPageToken": "CgkIChiAkceVjiYQ0b2SAQ",
"prevPageToken": "CgkIChDBwrK3mCYQ0b2SAQ",
"items": [
{
"kind": "blogger#post",
"id": "7706273476706534553",
"blog": {
"id": "2399953"
},
"published": "2011-08-01T19:58:00.000Z",
"updated": "2011-08-01T19:58:51.947Z",
"url": "http://buzz.blogger.com/2011/08/latest-updates-august-1st.html",
"selfLink": "https://www.googleapis.com/blogger/v3/blogs/2399953/posts/7706273476706534553",
"title": "Latest updates, August 1st",
"content": "elided for readability",
"author": {
"id": "401465483996",
"displayName": "Brett Wiltshire",
"url": "http://www.blogger.com/profile/01430672582309320414",
"image": {
"url": "http://4.bp.blogspot.com/_YA50adQ-7vQ/S1gfR_6ufpI/AAAAAAAAAAk/1ErJGgRWZDg/S45/brett.png"
}
},
"replies": {
"totalItems": "0",
"selfLink": "https://www.googleapis.com/blogger/v3/blogs/2399953/posts/7706273476706534553/comments"
}
},
{
"kind": "blogger#post",
"id": "6069922188027612413",
elided for readability
}
]
}
After I put it to the Json to Dart Convertor and the Model class I got was like this.
class PostList {
String kind;
String nextPageToken;
String prevPageToken;
List<Items> items;
PostList({this.kind, this.nextPageToken, this.prevPageToken, this.items});
PostList.fromJson(Map<String, dynamic> json) {
kind = json['kind'];
nextPageToken = json['nextPageToken'];
prevPageToken = json['prevPageToken'];
if (json['items'] != null) {
items = new List<Items>();
json['items'].forEach((v) {
items.add(new Items.fromJson(v));
});
}
}
Map<String, dynamic> toJson() {
final Map<String, dynamic> data = new Map<String, dynamic>();
data['kind'] = this.kind;
data['nextPageToken'] = this.nextPageToken;
data['prevPageToken'] = this.prevPageToken;
if (this.items != null) {
data['items'] = this.items.map((v) => v.toJson()).toList();
}
return data;
}
}
class Items {
String kind;
String id;
Blog blog;
String published;
String updated;
String url;
String selfLink;
String title;
String content;
Author author;
Replies replies;
Items(
{this.kind,
this.id,
this.blog,
this.published,
this.updated,
this.url,
this.selfLink,
this.title,
this.content,
this.author,
this.replies});
Items.fromJson(Map<String, dynamic> json) {
kind = json['kind'];
id = json['id'];
blog = json['blog'] != null ? new Blog.fromJson(json['blog']) : null;
published = json['published'];
updated = json['updated'];
url = json['url'];
selfLink = json['selfLink'];
title = json['title'];
content = json['content'];
author =
json['author'] != null ? new Author.fromJson(json['author']) : null;
replies =
json['replies'] != null ? new Replies.fromJson(json['replies']) : null;
}
Map<String, dynamic> toJson() {
final Map<String, dynamic> data = new Map<String, dynamic>();
data['kind'] = this.kind;
data['id'] = this.id;
if (this.blog != null) {
data['blog'] = this.blog.toJson();
}
data['published'] = this.published;
data['updated'] = this.updated;
data['url'] = this.url;
data['selfLink'] = this.selfLink;
data['title'] = this.title;
data['content'] = this.content;
if (this.author != null) {
data['author'] = this.author.toJson();
}
if (this.replies != null) {
data['replies'] = this.replies.toJson();
}
return data;
}
}
class Blog {
String id;
Blog({this.id});
Blog.fromJson(Map<String, dynamic> json) {
id = json['id'];
}
Map<String, dynamic> toJson() {
final Map<String, dynamic> data = new Map<String, dynamic>();
data['id'] = this.id;
return data;
}
}
class Author {
String id;
String displayName;
String url;
Image image;
Author({this.id, this.displayName, this.url, this.image});
Author.fromJson(Map<String, dynamic> json) {
id = json['id'];
displayName = json['displayName'];
url = json['url'];
image = json['image'] != null ? new Image.fromJson(json['image']) : null;
}
Map<String, dynamic> toJson() {
final Map<String, dynamic> data = new Map<String, dynamic>();
data['id'] = this.id;
data['displayName'] = this.displayName;
data['url'] = this.url;
if (this.image != null) {
data['image'] = this.image.toJson();
}
return data;
}
}
class Image {
String url;
Image({this.url});
Image.fromJson(Map<String, dynamic> json) {
url = json['url'];
}
Map<String, dynamic> toJson() {
final Map<String, dynamic> data = new Map<String, dynamic>();
data['url'] = this.url;
return data;
}
}
class Replies {
String totalItems;
String selfLink;
Replies({this.totalItems, this.selfLink});
Replies.fromJson(Map<String, dynamic> json) {
totalItems = json['totalItems'];
selfLink = json['selfLink'];
}
Map<String, dynamic> toJson() {
final Map<String, dynamic> data = new Map<String, dynamic>();
data['totalItems'] = this.totalItems;
data['selfLink'] = this.selfLink;
return data;
}
}
I removed All of those toJson Methods and renamed items
to posts
.
The Next thing we wanna do is to create A Function to Fetch those Blog Posts.
The One I wrote is
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Post List"),
centerTitle: true,
),
body: FutureBuilder(
// : null,
future: fetchPosts(),
builder: (context, snapshot) {
if (!snapshot.hasData) {
return Center(
child: CircularProgressIndicator(),
);
} else
return ListView.builder(
shrinkWrap: true,
itemCount: snapshot.data.posts.length ?? 1,
itemBuilder: (context, index) {
return Card(
child: ListTile(
title: Text(
snapshot.data.posts[index].title ?? "no items",
),
subtitle: Text(
snapshot.data.posts[index].author.displayName ??
"No Auther"),
),
);
},
);
}),
);
}
This will give you list of all the posts in your blog 🎉
If anything is unclear, feel free to check the source code at github.
If you have any suggestion or like to connect with me, you can find me on Twitter or can drop me a Mail at [email protected]. :blush:
Finally, if you found this helpful, please share this within your reach so that more people can benefit from this. And Follow me on Twitter for getting more posts like these 😉.